Conversation Component
This feature is in Maintenance mode. Nylas will patch bugs and vulnerabilities but is not currently working on new functionality.
View a list of threads or messages in a conversation view. Without writing a single line of code, the Conversation Component comes with:
- Theme options
- Chat through email
- Customization options
How It Works
The Conversation Component allows your users to send emails using a chat interface. When a new chat message is sent, an email is being sent. These will show in the user's mailbox.
Clean Conversations API
The Conversations Component uses the Clean Conversations endpoint. Contact the Nylas sales team or account manager for pricing. If the endpoint is not enabled, the Component will return an error message.
Quickstart
- Create a Conversation Component in your Nylas Dashboard.
- Add it to your application.
<head>
<!-- Import the script from CDN -->
<script src="https://unpkg.com/@nylas/components-conversation"></script>
</head>
<body>
<!-- Place the component on the page -->
<nylas-conversation id="<YOUR-COMPONENT-ID>"> </nylas-conversation>
</body>
That’s It! Your Conversation Component is ready to use!
Prerequisites
Before you use components, you must have the following prerequisites:
- A v2 Nylas application.
- The minimum required scopes:
- Agenda:
calendar
- Composer:
email.modify
,email.send
,contacts.read_only
- Contact list:
contacts.read_only
- Email:
email.modify
,contacts.read_only
- Messages:
email.read_only
,contacts.read_only
- Agenda:
- An application to add the component to.
Conversation Installation Options
You can install the Conversation Component 2 ways:
npm Package
- Run
npm i @nylas/components-conversation
oryarn add @nylas/components-conversation
- Then import and render the component.
// Import the web component
import '@nylas/components-conversation';
// In render method
<nylas-conversation></nylas-conversation>
Script Tag
Use the CDN script tag, https://unpkg.com/@nylas/components-conversation
.
<head>
<!-- Import the script from CDN -->
<script src="https://unpkg.com/@nylas/components-conversation"></script>
</head>
<body>
<!-- Place the component on the page -->
<nylas-conversation></nylas-conversation>
</body>
Ways to Use the Conversation Component
- Use the Conversation Component with Nylas Data - Choose this option if you want to integrate your existing Nylas accounts with the Conversation Component.
- Use the Conversation Component with External Data - Choose this option if you want to pass in your data.
Use the Conversation Component with Nylas Data
You'll learn how to use your Nylas accounts with the Conversation Component.
Create the Conversation Component
- Log in to your Nylas Dashboard.
- Click Components > Conversation
- Name your Component and select the account. To quickly start testing your Component select Enable for a single account.
- To learn how to enable components for multiple accounts see Authorizing Components.
- Use an existing access token or generate a new one.
- Enter the domain the component will run on. Without the domains the component will not run.
- To run on localhost, enter an asterisk.
*
. Remember to change this before going to production.
- To run on localhost, enter an asterisk.
- Save your new component.
- On the Edit page, you can configure your component behavior and appearance.
Generate an Access Token
Generating a new access token will not replace your existing access token; it will create a new access token with the same scopes as the previous token. You can revoke access tokens at any time.
Add the Component To Your Application
- On the Edit page, there are instructions for installing the new Component.
- If you haven't installed the Contact List Component already, open a terminal and run
npm i @nylas/components-conversation
. - Then add your Nylas Component. Copy and paste your component code into your application.
<nylas-conversation="your-component-id"></nylas-conversation>
. - You should refresh your application if needed. Any customization options set on the editing page will apply to your Component.
Use Conversation Component with External Data
You can pass in any data you want. It should be formatted as a Message or Thread object. Check out our sandbox for an example.
Authorizing Components
You can authorize components to work with a single account or multiple accounts.
Enable for a single account
Enabling components for a single account is a good way to get started quickly. If you use this method in your production application, you must authenticate each account from the Nylas Dashboard one-by-one.
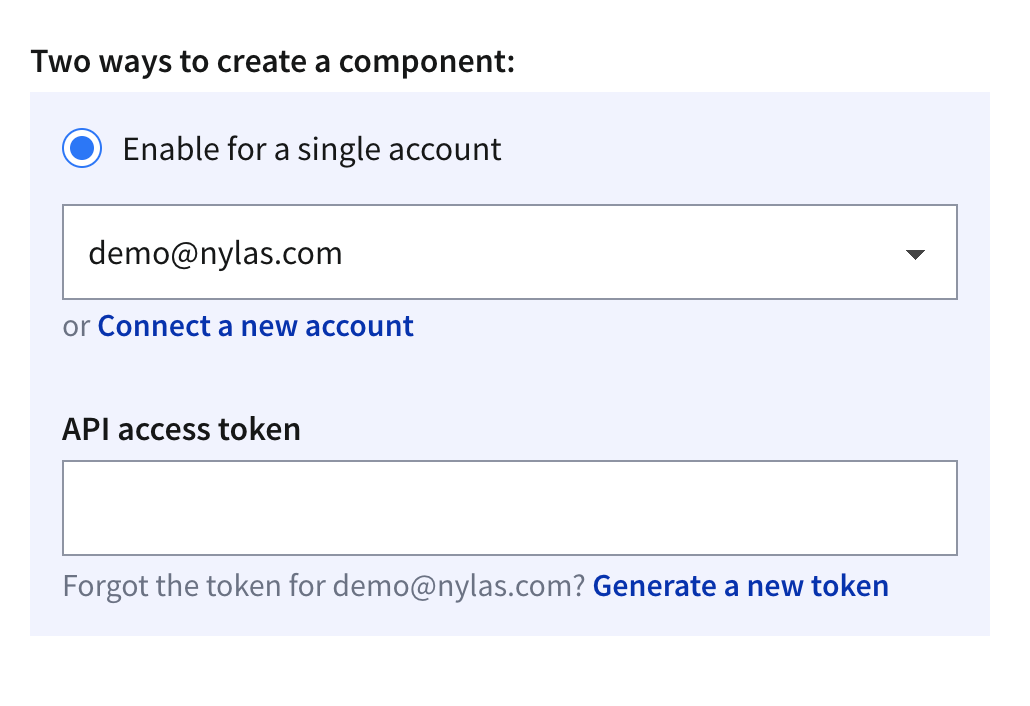
Enable for all accounts
🔍 Dashboard previews don't work when you enable components for all accounts.
To enable components for all accounts, you must pass in an end user's access token as a property of the component. One way to do this is to create a login screen, capture the access token, and pass it to the component. You can pass access_tokens
directly in the component, as in the following example.
<nylas-agenda id="COMPONENT-ID" access_token="USER-ACCESS-TOKEN"/>
Be sure to keep your end users' access tokens hidden, and never expose them online.
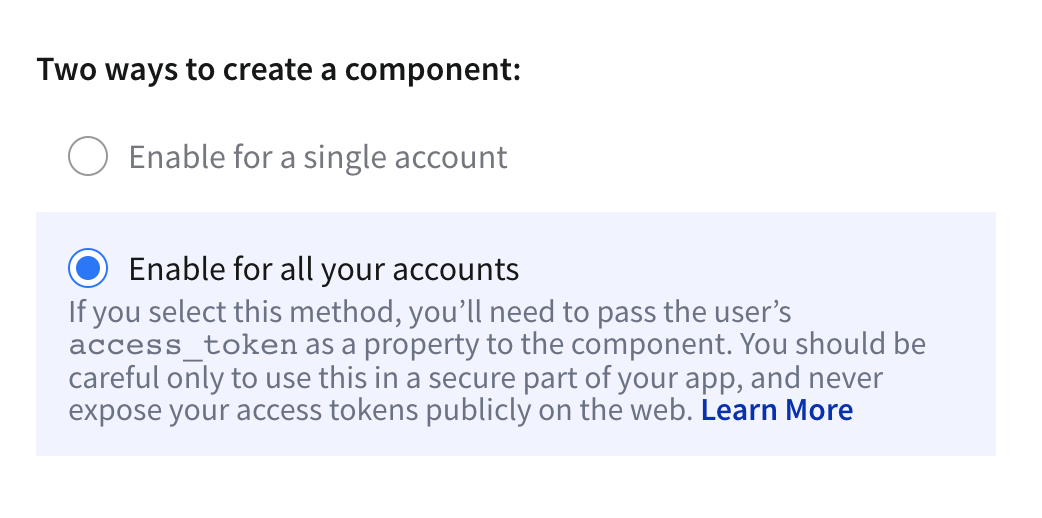
Customization
Nylas Components are flexible and can be customized for your needs.
Conversation Properties
Name | Type | Description | Default |
---|---|---|---|
thread_id | string | ID of the thread to display. | None |
messages | array | Array of message objects | None |
theme | string | theme-1 , theme-2 , theme-3 , gmail , ellsworth-kelly |
theme-1 |
show_avatars | boolean | Show contact avatars | true |
show_reply | boolean | Show replies to messages | true |
Conversation Event Listeners
Name | Description |
---|---|
manifestLoaded |
When the event manifest is loaded. The handler is passed the manifest argument. |
onError |
Emitted if an error occurs while fetching data in the component. The handler is passed an object with the component id as the key and the error as the value. |
sendMessageClicked |
When the send message button is clicked. |
Allowed Domains
You can restrict the domains that your component works on using glob patterns, as in the following examples:
nylas.com
matches onlynylas.com
.subdomain.nylas.com
matches onlysubdomain.nylas.com
.*subdomain.nylas.com
matchessubdomain.nylas.com
andsub.sub.subdomain.nylas.com
.*
matches any domain.
Domain restriction applies only to the domain name. You cannot restrict URL paths (for example, developer.nylas.com/docs/
) or protocols (for example, HTTPS).
How Properties Are Handled
Nylas Components follow an order of operations to decide if the dashboard or code takes precedence.
- If the property is passed directly to the Component, use that first.
- If the property is not passed directly in the Component, use the dashboard settings.
- If there are no properties set in the Component or the dashboard, use the Component defaults.
Demo Apps
What's Next?
Take a look at other Components:
- Mailbox Component
- Contact List Component
- Agenda Component
- Conversation Component
- Email Component
- Composer Component
Check us out on GitHub: