Mailbox Component
This feature is in Maintenance mode. Nylas will patch bugs and vulnerabilities but is not currently working on new functionality.
Use the Nylas Mailbox Component to connect to any inbox and provide a custom front end for your applications.
Without needing to write a single line of code, the Mailbox Component comes with:
- To, from, subject display
- Message previews
- Thread display and expansion on click
- Ability to star threads
- Ability to mark threads as read or unread
- Thread selection, including selecting all threads
- Built-in pagination
- Customization options
Quickstart
- Create a Mailbox component in your Nylas Dashboard.
- Add it to your application.
<head>
<!-- Import the script from CDN -->
<script src="https://unpkg.com/@nylas/components-mailbox"></script>
</head>
<body>
<!-- Place the component on the page -->
<nylas-mailbox id="<PASTE_YOUR_COMPONENT_ID>"></nylas-mailbox>
</body>
That’s It! Your Mailbox Component is ready to use!
Prerequisites
Before you use components, you must have the following prerequisites:
- A v2 Nylas application.
- The minimum required scopes:
- Agenda:
calendar
- Composer:
email.modify
,email.send
,contacts.read_only
- Contact list:
contacts.read_only
- Email:
email.modify
,contacts.read_only
- Messages:
email.read_only
,contacts.read_only
- Agenda:
- An application to add the component to.
Mailbox Installation Options
You can install the Mailbox Component 2 ways:
npm Package
- Run
npm i @nylas/components-mailbox
oryarn add @nylas/components-mailbox
- Then import and render the component.
// Import the web component
import '@nylas/components-mailbox';
// In render method
<nylas-mailbox></nylas-mailbox>
Script Tag
Use the CDN script tag, https://unpkg.com/@nylas/components-mailbox
.
<head>
<!-- Import the script from CDN -->
<script src="https://unpkg.com/@nylas/components-mailbox"></script>
</head>
<body>
<!-- Place the component on the page -->
<nylas-mailbox></nylas-mailbox>
</body>
Ways to Use the Mailbox Component
- Use the Mailbox Component with Nylas Data - Choose this option if you want to integrate your Component Nylas accounts with the Mailbox Component.
- Use the Mailbox Component with External Data - Choose this option if you want to bring your own data to the Component.
Use the Mailbox Component with Nylas Data
You'll learn how to use your Nylas accounts with the Mailbox Component.
Create the Mailbox Component
- Log in to your Nylas dashboard.
- Click Components > Mailbox
- Name your Component and select the account. To quickly start testing your Component select Enable for a single account.
- To learn how to enable components for multiple accounts see Authorizing Components
- Use an existing access token or generate a new one.
- Enter the domain the component will run on. Without the domain, the component will not run.
- To run on localhost, enter an asterisk.
*
. Remember to change this before going to production.
- To run on localhost, enter an asterisk.
- Save your new component.
- On the Edit page, you can configure your component behavior and appearance.
Generate an Access Token
Generating a new access token will not replace your existing access token; it will create a second access token with the same scopes as the previous token. You can revoke access tokens at any time.
Add the Component To Your Application
- On the Edit page, there are instructions for installing the new component.
- We recommend that you filter or query your threads. Mailbox will display threads located in inbox and trash.
- Search will accept any keyword and filter accepts any query parameter listed for Threads.
- If you haven't installed the Mailbox Component already, open a terminal and run
npm i @nylas/components-mailbox
. - Then add your Nylas component. Copy and paste your component code into your application.
<nylas-mailbox id="your-component-id" ></nylas-mailbox>
- You should refresh your application if needed. Any configuration options set on the editing page will apply to your Component.
Use the Mailbox Component with External Data
You can pass your own thread data for Mailbox to display. The threads format and type need to match the Threads object.
<script>
import Mailbox from "@nylas/components-mailbox";
const staticThreads = [
{
account_id: "3aols9hb9fkqtso7zkzcmwgwv",
drafts: [],
first_message_timestamp: 1634148124,
has_attachments: false,
id: "5mr2pufvjqgfcj3p0x2jp0d27",
labels: [
{
display_name: "IMPORTANT",
id: "8l6c4d11y1p4dm4fxj52whyr9",
name: "important"
},
{
display_name: "SENT",
id: "41nxdpa2klw8u0ml0c4pmmi9q",
name: "sent"
},
{
display_name: "INBOX",
id: "d9zkcr2tljpu3m4qpj7l2hbr0",
name: "inbox"
}
],
last_message_received_timestamp: 1634148124,
last_message_sent_timestamp: 1634149824,
last_message_timestamp: 1634149824,
messages: [
{
account_id: "3aols9hb9fkqtso7zkzcmwgwv",
bcc: [],
cc: [],
date: 1634148124,
files: [],
from: [
{
email: "healthcare.demo@nylas.com",
name: "Rebecca Lee Crumpler"
}
],
id: "2oxbhesmhekucib22500nzsnj",
labels: [
{
display_name: "IMPORTANT",
id: "8l6c4d11y1p4dm4fxj52whyr9",
name: "important"
},
{
display_name: "INBOX",
id: "d9zkcr2tljpu3m4qpj7l2hbr0",
name: "inbox"
}
],
object: "message",
reply_to: [],
snippet:
"Hi Dorothy, Want to get dinner Wednesday night? I'm free 5-9 pm?",
starred: false,
subject: "Dinner Wednesday? 😺",
thread_id: "5mr2pufvjqgfcj3p0x2jp0d27",
to: [
{
email: "demo@nylas.com",
name: "Dorothy Vaughan"
}
],
unread: false
},
{
account_id: "3aols9hb9fkqtso7zkzcmwgwv",
bcc: [],
cc: [],
date: 1634149824,
files: [],
from: [
{
email: "demo@nylas.com",
name: "Dorothy Vaughan"
}
],
id: "enppimg7p0udaoydjzkep95zo",
labels: [
{
display_name: "SENT",
id: "41nxdpa2klw8u0ml0c4pmmi9q",
name: "sent"
}
],
object: "message",
reply_to: [],
snippet:
"Yes, let's make a plan! On Wed, Oct 13, 2021 at 8:02 AM Rebecca Lee Crumpler <healthcare.demo@nylas.com> wrote: Hi Dorothy, Want to get dinner Wednesday night? I'm free 5-9 pm?",
starred: false,
subject: "Re: Dinner Wednesday? 😺",
thread_id: "5mr2pufvjqgfcj3p0x2jp0d27",
to: [
{
email: "healthcare.demo@nylas.com",
name: "Rebecca Lee Crumpler"
}
],
unread: false
}
],
object: "thread",
participants: [
{
email: "demo@nylas.com",
name: "Dorothy Vaughan"
},
{
email: "healthcare.demo@nylas.com",
name: "Rebecca Lee Crumpler"
}
],
snippet:
"Yes, let's make a plan! On Wed, Oct 13, 2021 at 8:02 AM Rebecca Lee Crumpler <healthcare.demo@nylas.com> wrote: Hi Dorothy, Want to get dinner Wednesday night? I'm free 5-9 pm?",
starred: false,
subject: "Dinner Wednesday? 😺",
unread: false,
version: 119
}
];
</script>
<style>
main {
font-family: sans-serif;
text-align: center;
height: 500px;
}
iframe {
display: none !important;
}
</style>
<main>
<h1>Nylas Mailbox</h1>
<nylas-mailbox all_threads={staticThreads}>
</main>
Authorizing Components
You can authorize components to work with a single account or multiple accounts.
Enable for a single account
Enabling components for a single account is a good way to get started quickly. If you use this method in your production application, you must authenticate each account from the Nylas Dashboard one-by-one.
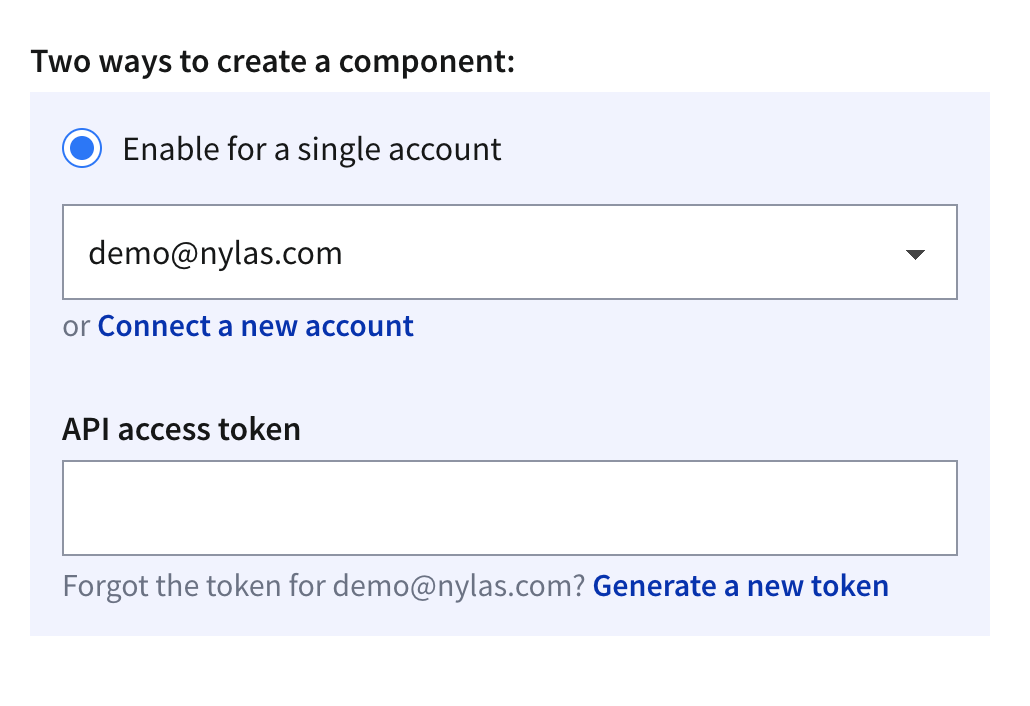
Enable for all accounts
🔍 Dashboard previews don't work when you enable components for all accounts.
To enable components for all accounts, you must pass in an end user's access token as a property of the component. One way to do this is to create a login screen, capture the access token, and pass it to the component. You can pass access_tokens
directly in the component, as in the following example.
<nylas-agenda id="COMPONENT-ID" access_token="USER-ACCESS-TOKEN"/>
Be sure to keep your end users' access tokens hidden, and never expose them online.
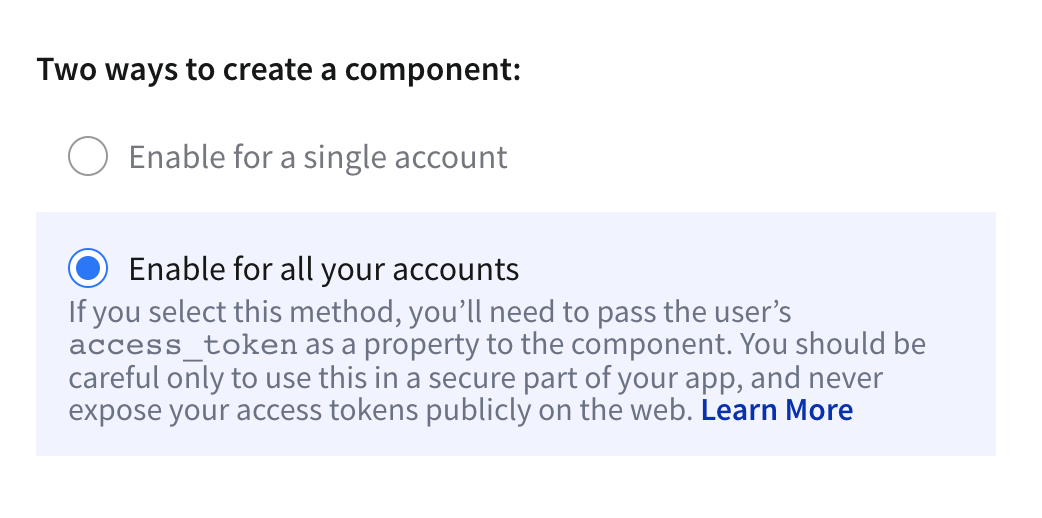
Administrator Requirements
The Mailbox Component requires administrative privileges in order to authorize all accounts from the dashboard. Non-administrative users can only authorize a single account.
Customization
Nylas Components are flexible and can be customized for your needs.
Mailbox Properties
Name | Type | Description | Default |
---|---|---|---|
show_thread_checkbox | boolean | Choose whether or not to display selection checkboxes beside each thread. | True |
items_per_page | number | The number of threads to show per page. | 13 |
show_star | boolean | Choose whether or not stars appear on each thread. | false |
header | string | Change what title is displayed at the top of mailbox. | Null or not displayed |
keyword_to_search | string | Return threads that match any string | None |
query_string | string | Search for messages using query strings and display those in the component. "in=trash from=kat@spacetech.com" Allowed parameters. |
None |
show_reply | boolean | Show reply field | false |
show_reply_all | boolean | Show reply all field | false |
show_forward | boolean | Show forward field | false |
theme | string | Set the custom theme. Possible values are auto , light , dark or URL / relative path to custom CSS file. |
auto |
unread_status [Deprecated] | string | Deprecated as of v1.1.0 - Use the passed thread object's unread prop insteadread , unread , or default . If set to default , uses the read status attached to the thread/message. Otherwise sets all threads and messages in the mailbox to be read and unread for read and unread respectively. |
default |
Mailbox Event Listeners
Use event listeners to customize the mailbox.
Name | Description |
---|---|
manifestLoaded |
This event is dispatched on load when the manifest is loaded. The handler is passed the manifest argument. |
onError |
Emitted if an error occurs while fetching data in the component. The handler is passed an object with the component id as the key and the error as the value. |
threadClicked |
When a thread is opened or closed. The handler is passed the event and selectedThreads arguments. |
onChangeSelectedReadStatus |
When selected emails or threads are marked as read or unread. The handler is passed the event and selectedThreads arguments. |
onDeleteSelected |
When emails or threads are deleted. If a single email or thread is deleted, it is captured in thread . If multiple emails or threads are deleted, it is captured in selectedThreads . The handler is passed the event and selectedThreads arguments. |
onStarSelected |
When selected emails or threads are starred or unstarred. The handler is passed the event and selectedThreads arguments. |
refreshClicked |
When the refresh button in the mailbox is clicked. The handler is passed the event argument. |
onSelectOneClicked |
When a single email or thread is selected by clicking on the checkbox. The handler is passed the event and thread arguments |
onSelectAllClicked |
When select all checkbox is checked or unchecked. The handler is passed the event and selectedThreads arguments. |
returnToMailbox |
When back button is clicked after clicking a thread. |
Custom CSS
To customize the CSS, you can change the theme
custom property to one of the provided themes by Nylas:
<nylas-mailbox id="NYLAS_MAILBOX_COMPONENT_ID" theme="light"></nylas-mailbox>
You can also easily override styles for pre-selected themes by writing your own CSS vars. The customizable CSS variables for Mailbox Component are the following and the value assigned should be a valid CSS property value:
.nylas-mailbox {
/* Mailbox variables */
--nylas-mailbox-font-family: sans-serif;
--nylas-mailbox-border-color: #e1e1e1;
--nylas-mailbox-vertical-border-color: #e1e1e1;
--nylas-mailbox-horizontal-border-color: #e1e1e1;
--nylas-mailbox-hover-shadow-color: #cacaca;
--nylas-mailbox-header-background: #fff;
--nylas-mailbox-toolbar-background: #fff;
--nylas-mailbox-read-background: #f5f5f5;
--nylas-mailbox-unread-background: #fff;
--nylas-mailbox-checked-border-color: #4169e1;
--nylas-mailbox-checked-bg-color: #e0e6f9;
--nylas-mailbox-checkedbox-active-color: #4169e1;
--nylas-mailbox-pagination-button-color: #4b4b4b;
--nylas-mailbox-pagination-button-background: #fbfcfe;
--nylas-mailbox-pagination-button-background-disabled: #eaeaea;
--nylas-mailbox-pagination-button-border-color: #eaeaea;
--nylas-mailbox-pagination-button-hover-color: #eaeaea;
--nylas-mailbox-pagination-indicator-color: #2c2c2c;
}
You can also provide your own stylesheet to the theme
custom property. Review the light.css theme to get the CSS variable names:
<nylas-mailbox
id="NYLAS_MAILBOX_COMPONENT_ID"
show_header="false"
theme="https://example.com/my-mailbox-theme.css"
></nylas-mailbox>
Allowed Domains
You can restrict the domains that your component works on using glob patterns, as in the following examples:
nylas.com
matches onlynylas.com
.subdomain.nylas.com
matches onlysubdomain.nylas.com
.*subdomain.nylas.com
matchessubdomain.nylas.com
andsub.sub.subdomain.nylas.com
.*
matches any domain.
Domain restriction applies only to the domain name. You cannot restrict URL paths (for example, developer.nylas.com/docs/
) or protocols (for example, HTTPS).
How Properties Are Handled
Nylas Components follow an order of operations to decide if the dashboard or code takes precedence.
- If the property is passed directly to the Component, use that first.
- If the property is not passed directly in the Component, use the dashboard settings.
- If there are no properties set in the Component or the dashboard, use the Component defaults.
Demo Apps
What's Next?
Take a look at other Components:
- Mailbox Component
- Contact List Component
- Agenda Component
- Conversation Component
- Email Component
- Composer Component
Check us out on GitHub: