Email Component
This feature is in Maintenance mode. Nylas will patch bugs and vulnerabilities but is not currently working on new functionality.
Display a single email or thread using the Email Component. Without needing to write a single line of code, the Email Component comes with:
- Theme options
- To, from, subject display
- Thread display and expansion on click
- Ability to star threads
- Ability to mark threads as read or unread
- Customization options
Quickstart
- Create an Email component in your Nylas Dashboard.
- Add it to your application.
<head>
<!-- Import the script from CDN -->
<script src="https://unpkg.com/@nylas/components-email"></script>
</head>
<body>
<!-- Place the component on the page -->
<nylas-email id="<PASTE_YOUR_COMPONENT_ID>"></nylas-email>
</body>
That’s It! Your Email Component is ready to use!
Prerequisites
Before you use components, you must have the following prerequisites:
- A v2 Nylas application.
- The minimum required scopes:
- Agenda:
calendar
- Composer:
email.modify
,email.send
,contacts.read_only
- Contact list:
contacts.read_only
- Email:
email.modify
,contacts.read_only
- Messages:
email.read_only
,contacts.read_only
- Agenda:
- An application to add the component to.
Email Installation Options
You can install the Email Component 2 ways:
npm Package
- Run
npm i @nylas/components-email
oryarn add @nylas/components-email
- Then import and render the component.
// Import the web component
import '@nylas/components-email';
// In render method
<nylas-email></nylas-email>
Script Tag
Use the CDN script tag, https://unpkg.com/@nylas/components-email
.
<head>
<!-- Import the script from CDN -->
<script src="https://unpkg.com/@nylas/components-email"></script>
</head>
<body>
<!-- Place the component on the page -->
<nylas-email></nylas-email>
</body>
Ways to Use the Email Component
- Use the Email Component with Nylas Data - Choose this option if you want to integrate your existing Nylas accounts with the Email Component.
- Use the Email Component with External Data - Choose this option if you want to pass in your data.
Use the Email Component with Nylas Data
You'll learn how to use your Nylas accounts with the Email Component.
Create the Email Component
- Log in to your Nylas Dashboard.
- In your Components tab, click Email.
- Name your Component and select the account. To quickly start testing your Component select Enable for a single account.
- To learn how to enable components for multiple accounts see Authorizing Components.
- Use an existing access token or generate a new one.
- Enter the domain the component will run on. Without the domains the component will not run.
- To run on localhost, enter an asterisk.
*
. Remember to change this before going to production.
- To run on localhost, enter an asterisk.
- Save your new component.
- On the Edit page, you can configure your component behavior and appearance.
Generate an Access Token
Generating a new access token will not replace your existing access token; it will create a second access token with the same scopes as the previous token. You can revoke access tokens at any time.
Add the Component To Your Application
Before you add the Component to your application, you can either choose a thread ID in the dashboard or you can pass in a message ID or thread ID directly into the Email Component.
Dashboard Thread ID
- On the Edit page, there are instructions for installing the new component.
- Make sure to select which Thread to view.
- If you haven't installed the Email Component already, open a terminal and run
npm i @nylas/components-email
. - Then add your Nylas component. Copy and paste your component code into your application.
<nylas-email id="your-email-id" > </nylas-email>
- You should refresh your application if needed. Any configuration options set on the editing page will apply to your Component.
API Message and Thread ID
-
On the Edit page, there are instructions for installing the new component.
-
If you haven't installed the Email Component already, open a terminal and run
npm i @nylas/components-email
. -
Then add your Nylas component. Copy and paste your component code into your application.
<nylas-email id="your-email-id" > </nylas-email>
-
Leave Thread to view empty.
-
Then either pass in a thread or message ID.
<nylas-email id="<NYLAS_COMPONENT_ID>" thread_id="<THREAD_ID"></nylas-email>
//or
<nylas-email id="<NYLAS_COMPONENT_ID>" message_id="<MESSAGE_ID>"></nylas-email> -
You should refresh your application if needed. Any configuration options set on the editing page will apply to your Component.
Use Email Component with External Data
You'll learn how to pass threads and messages to display an email thread within your application.
- Install the Nylas Email Component.
npm i @nylas/components-email
.
The Nylas Email Component can accept a Nylas Thread object or a Nylas Message object.
You can pass in:
thread
- A thread of one or more messagesmessage
- A single message
Authorizing Components
You can authorize components to work with a single account or multiple accounts.
Enable for a single account
Enabling components for a single account is a good way to get started quickly. If you use this method in your production application, you must authenticate each account from the Nylas Dashboard one-by-one.
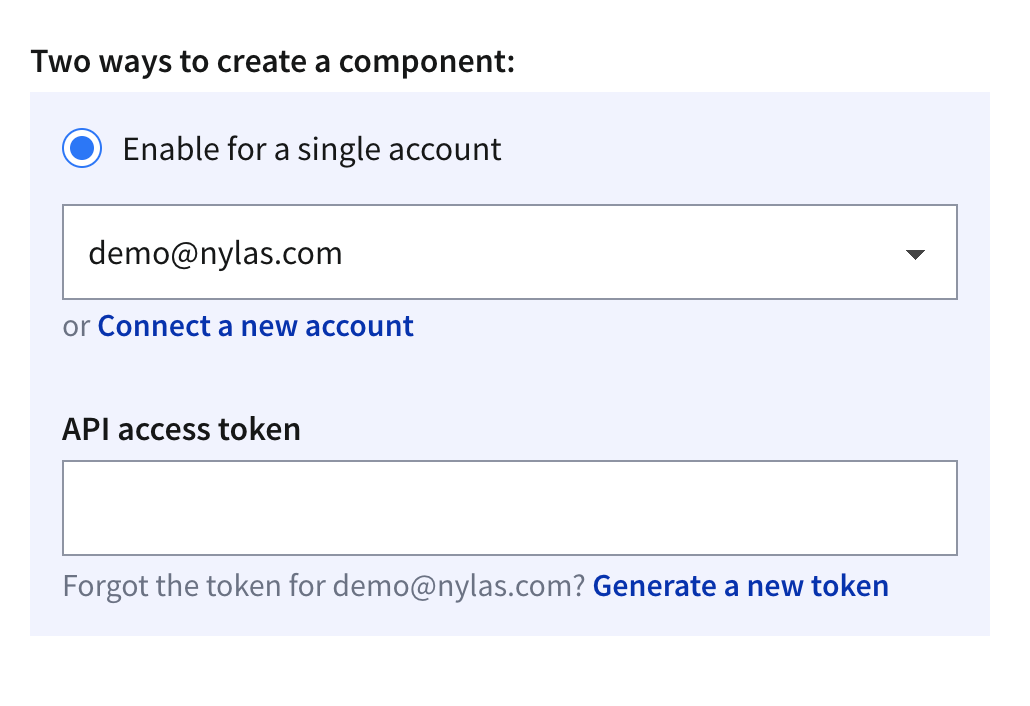
Enable for all accounts
🔍 Dashboard previews don't work when you enable components for all accounts.
To enable components for all accounts, you must pass in an end user's access token as a property of the component. One way to do this is to create a login screen, capture the access token, and pass it to the component. You can pass access_tokens
directly in the component, as in the following example.
<nylas-agenda id="COMPONENT-ID" access_token="USER-ACCESS-TOKEN"/>
Be sure to keep your end users' access tokens hidden, and never expose them online.
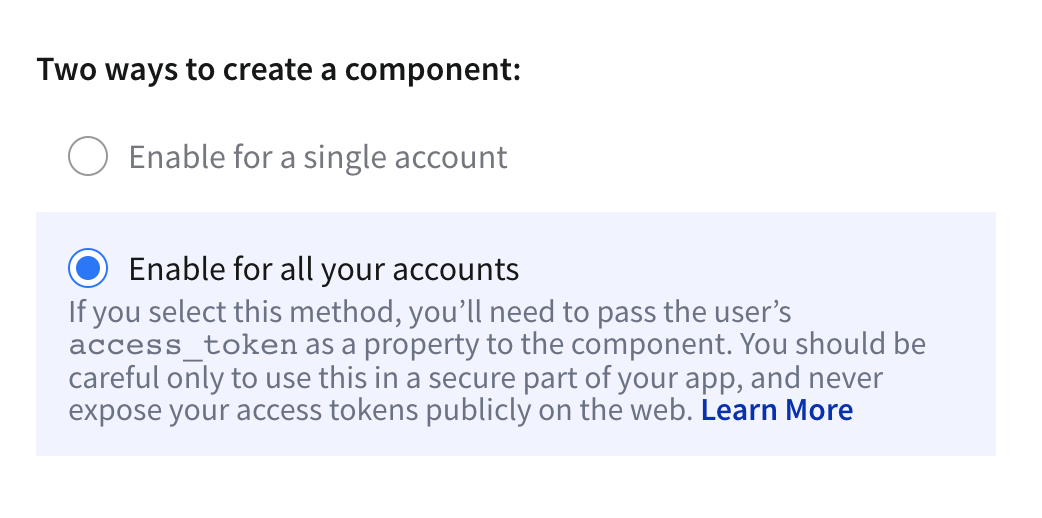
Customization
Nylas Components are flexible and can be customized for your needs.
Email Properties
Name | Type | Description |
---|---|---|
clean_conversation |
boolean | The Email Component utilizes our Clean Conversations endpoint to remove attachments, images, and other extra information from emails. |
click_action |
string | default or custom . Set the behaviour when clicking on an Email. If default is selected, will close the Email if it's an expanded thread, expand the Email if it's a closed thread, and set the active thread to read if previously unread. |
message_id |
string | Pass in a message_id . |
message |
Message | An external Nylas Message object. |
show_contact_avatar |
boolean | Show contact pictures |
show_expanded_email_view_onload |
boolean | When the messages are loaded, expand them on page load. |
show_forward |
boolean | Choose if the forward button will appear on each thread. |
show_number_of_messages |
boolean | Display or hide the number of messages in a thread when collapsed. |
show_received_timestamp |
boolean | Show or hide the timestamp of a message. |
show_reply |
boolean | Show or hide the reply button on each thread. |
show_reply_all |
boolean | Show or hide the reply all button on each thread. |
show_star |
boolean | Show or hide the star button on each thread. |
show_thread_actions |
boolean | Show delete and unread icons. |
theme |
string | Set the custom theme. Possible values are auto , light , dark or URL / relative path to custom CSS file. |
thread_id |
string | Pass in a thread_id . If using this option, leave the ID blank in the dashboard. |
thread |
Thread | An external Nylas Thread object of one or more messages. |
unread [Deprecated] |
boolean | Deprecated as of v1.1.0 - Use the passed thread/message object's unread prop insteadIf set to true, sets all messages in thread to unread (visually). If false, sets all messages in thread to read (visually). If set to null , uses the read status attached to the thread. |
Event Listeners
Use event listeners to customize the mailbox.
Name | Description |
---|---|
manifestLoaded |
This event is dispatched on load when the manifest is loaded. The handler is passed the manifest argument. |
threadLoaded |
This event is dispatched on load when the email thread is loaded. The handler is passed the thread object loaded. |
messageLoaded |
This event is dispatched on load when the email message is loaded. The handler is passed the message object loaded. |
onError |
Emitted if an error occurs while fetching data in the component. The handler is passed an object with the component id as the key and the error as the value. |
threadClicked |
When an email is opened, closed, expanded, or condensed. The handler is passed the event , thread , and messageType . |
toggleThreadUnreadStatus |
When is email is marked as read or unread. The handler is passed the event and thread . |
threadDeleted |
When a thread or email is deleted. The handler is passed the event and thread . |
threadStarred |
When an email is starred or unstarred. The handler is passed the event and thread . |
messageClicked |
When an email is expanded and an individual message is clicked. The handler is passed the event , thread , and the clicked draft message . |
draftClicked |
When an individual draft message in the thread is clicked. The handler is passed the event , thread , and the clicked message . |
returnToMailbox |
When the left arrow at the top of the email header is clicked. The event is only dispatched when click_action="mailbox" . The handler is passed the event and thread . |
replyClicked |
Emitted when reply button is clicked. The handler is passed the event , thread , the clicked message and value of a created message for reply. |
replyAllClicked |
Emitted when reply all button is clicked. The handler is passed the event , thread , the clicked message and value of a created message for reply all. |
forwardClicked |
Emitted when forward button is clicked. The handler is passed the event , thread , the clicked message and value of a created message for forward. |
downloadClicked |
Emitted when an attachment or a file in the message body is downloaded. The handler is passed the event , thread and file . |
fileClicked |
Emitted when a file attachment is clicked. The handler is passed the event and file . |
Custom CSS
To customize the CSS, you can change the theme
custom property to one of the provided themes by Nylas:
<nylas-email id="NYLAS_EMAIL_COMPONENT_ID" theme="light"></nylas-email>
You can also easily override styles for pre-selected themes by writing your own CSS vars. The customizable CSS variables for Email Component are the following and the value assigned should be a valid CSS property value:
.nylas-email {
/* Email variables */
--nylas-email-background: #f5f5f5;
--nylas-email-unread-background: #fff;
--nylas-email-body-background: #fff;
--nylas-email-header-background: #fff;
--nylas-email-border-style: #dfe1e8;
--nylas-email-border-style-expanded: #e1e1e1;
--nylas-email-snippet-color: #6e6e6e;
--nylas-email-subject-color: #4b4b4b;
--nylas-email-participant-color: #4b4b4b;
--nylas-email-thread-message-count-color: #2c2c2c;
--nylas-email-body-color: #2c2c2c;
--nylas-email-unread-subject-color: #2c2c2c;
--nylas-email-unread-thread-message-count-color: #4169e1;
--nylas-email-message-date-color: #8e8e8e;
--nylas-email-message-to-color: #8e8e8e;
--nylas-email-message-from-color: var(--nylas-email-body-color);
--nylas-email-draft-label-color: #cc4841;
--nylas-email-action-icons-color: #4b4b4b;
--nylas-email-button-hover-background: #e0e6f9;
--nylas-email-message-border-color: #eaeaea;
--nylas-email-star-button-inactive-color: #b3b3b3;
--nylas-email-star-button-disabled-color: #eaeaea;
--nylas-email-star-button-active-color: #ffc107;
--nylas-email-star-button-hover-color: #f7c90b;
--nylas-email-attachment-border-style: #cacaca;
--nylas-email-attachment-button-color: var(--nylas-email-snippet-color);
--nylas-email-attachment-button-bg: #fff;
--nylas-email-attachment-button-hover-bg: #f5f5f5;
}
You can also provide your own stylesheet to the theme
custom property. Review the light.css theme to get the CSS variable names:
<nylas-email
id="NYLAS_EMAIL_COMPONENT_ID"
show_header="false"
theme="https://example.com/my-email-theme.css"
></nylas-email>
Allowed Domains
You can restrict the domains that your component works on using glob patterns, as in the following examples:
nylas.com
matches onlynylas.com
.subdomain.nylas.com
matches onlysubdomain.nylas.com
.*subdomain.nylas.com
matchessubdomain.nylas.com
andsub.sub.subdomain.nylas.com
.*
matches any domain.
Domain restriction applies only to the domain name. You cannot restrict URL paths (for example, developer.nylas.com/docs/
) or protocols (for example, HTTPS).
How Properties Are Handled
Nylas Components follow an order of operations to decide if the dashboard or code takes precedence.
- If the property is passed directly to the Component, use that first.
- If the property is not passed directly in the Component, use the dashboard settings.
- If there are no properties set in the Component or the dashboard, use the Component defaults.
Demo Apps
What's Next?
Take a look at other Components:
- Mailbox Component
- Contact List Component
- Agenda Component
- Conversation Component
- Email Component
- Composer Component
Check us out on GitHub: