Production Checklist
Integrating with Nylas is a straightforward process, but there are key considerations you must make depending on your specific use case. This guide provides a comprehensive checklist of all the essential components every Nylas integration needs. After completing this guide, you can feel comfortable knowing that you've added rock-solid features to your app that are ready to deploy to production with minimal friction.
The basic steps to get started are:
- Sign up for your free account to create a new Nylas organization.
- Nylas creates a new app when you first login to your account, follow our guide to get your developer API keys to get the Client ID and Client Secret for your Nylas app. You'll need these to authenticate user accounts.
- Decide if you will use the Nylas Python, Java, Node.js / JavaScript, or Ruby SDK. Or if you will use a separate third-party HTTP library.
- Authenticate an account using Nylas Hosted Auth or Native Auth to generate an access token for the user account. Store this access token securely on your backend infrastructure. Nylas will begin syncing the account the moment it's connected.
- Use the account access token as a bearer token to make requests for user data and functionality via the Nylas Email, Calendar, and Contacts APIs.
You should also consider the following when building your integration, all of these are covered in this guide:
- Webhooks - If you want to watch for changes to your user's email inbox, calendar, or contacts book, you'll need to setup a scalable webhook listener and queue manager to handle incoming changes.
- Access Token Management - Your app needs to securely store user access tokens to protect user data.
- User Data Storage - You might want to store user data in your own database to access it without making repeated requests to Nylas.
- Error Handling & Monitoring - Changes to third-party user accounts can cause authorization issues that you'll need to account for in your app design.
Application Diagram
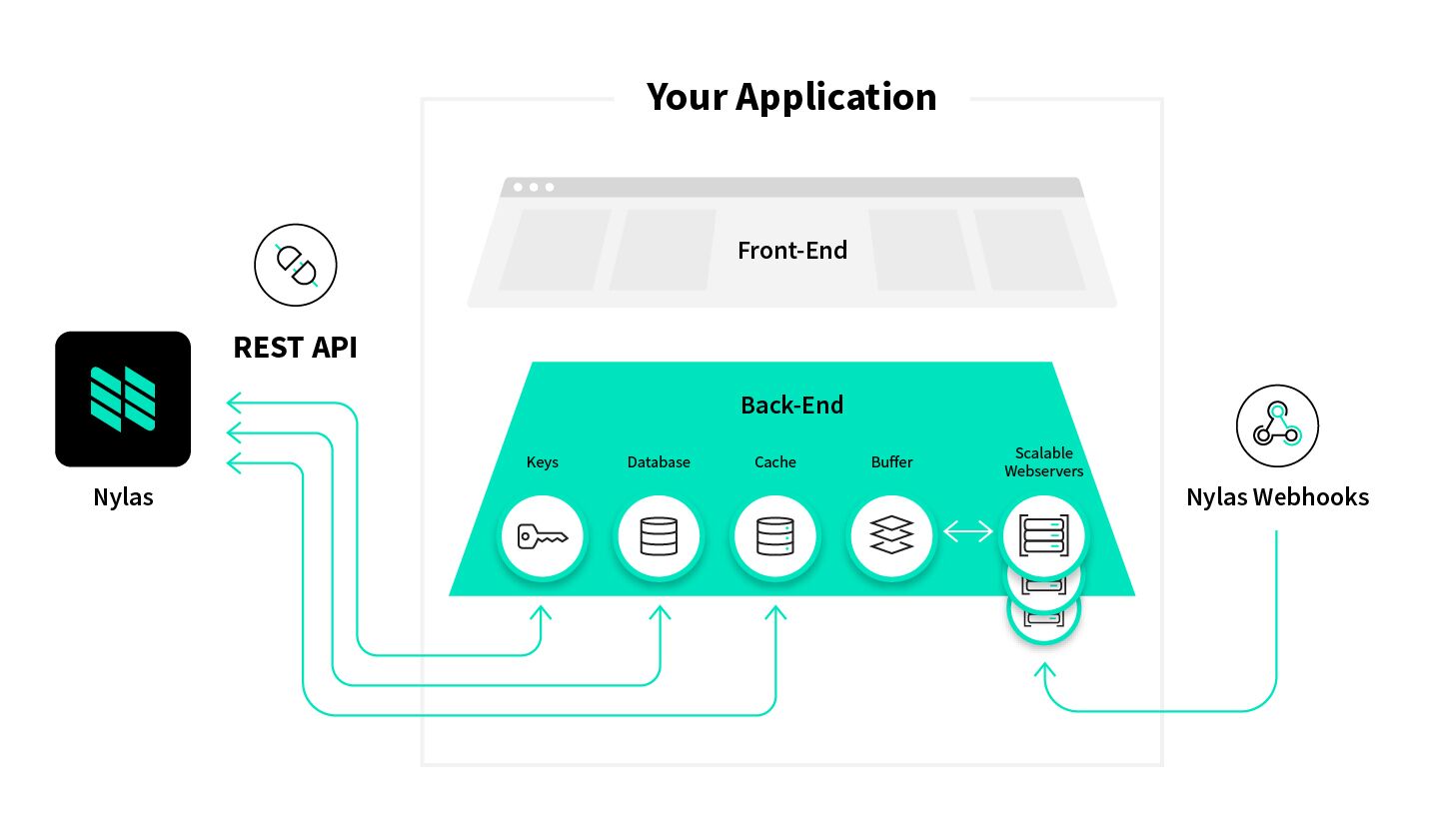
Create Your Organization
An organization in the Nylas platform is how we identify your company. Billing, support, data usage, and feature enablement are handled at the organization level.
When you first sign up with Nylas, you’ll be on an unpaid trial. Nylas trial environments are almost identical to production, with only a few differences:
- You are limited to 10 linked accounts.
- Message tracking features (analytics) are turned off for trial accounts. If you need to test message tracking, contact your Nylas sales representative.
This means that you can complete a full production-ready integration during your trial/POC, then start authenticating accounts in production without any changes. When you become a paid customer, this organization will be your production organization, so protect passwords and secrets.
Set Up Your Nylas Apps
Nylas applications are a good way to separate your development, staging, and production environments inside a Nylas organization. Each application has a unique client ID and secret. The client ID and secret are used to manage and authenticate accounts, but don’t provide access to data for accounts you authenticate. However, these should still be treated just like any other credentials, since they allow account and application management. Don't store them in your source code or unencrypted storage, and follow other security best practices.
Each Application has separate configurations for:
- Webhooks - You can enable webhooks with specific triggers for development applications to test them before enabling them on your production app. This is useful because the volume of webhooks on a production application with many accounts can be too high for effective testing.
- Custom Authentication - If you’re using Hosted Authentication, you might want to customize this process with your company’s information and logo. If you have multiple products you can have an application for each one, each with a different customized experience.
- Google & O365 OAuth credentials - Different environments should have different Microsoft 365 or Google Cloud applications so that you aren’t using a production app to test.
* This is critical to ensure continued testing access for Google accounts. When you submit a Google project application for verification, it disables any sensitive or restricted scopes you had enabled until your app is approved, which could block development. - API Version - Nylas uses API versioning to ensure we don't impact customers' production applications when we release new features or potentially breaking changes, see API Versioning for more details.
Choose Your SDK or HTTP Library
If your language doesn’t have a Nylas SDK, we recommend an HTTP library. Interactions with Nylas will still be straightforward, because Nylas is a standard REST API that accepts and returns JSON objects on every endpoint.
Choose an Authentication Method
One of the key values Nylas provides is offloading the burden of authenticating different types of email accounts securely and easily for end-users. We offer two different ways to authenticate accounts based on your application’s requirements: hosted (Nylas handles the whole process) and native authentication (full customization with authentication information submitted to Nylas).
Nylas Hosted Auth
Nylas hosted authentication is the recommended method to implement authentication because it offloads the entire process to Nylas, allowing you to get to market quicker. It handles the entire authentication flow, including provider detection, server settings detection, OAuth, Single sign-on, credential collection, and credential storage. Nylas also offers options to customize the hosted authentication experience to brand it as your own.
Here’s the basic flow of Nylas hosted authentication:
- You create a custom URL that redirects your users to Nylas hosted authentication.
- The user clicks the link, then signs in to their account, and gives your app permission to access their account with the requested scopes.
- After authenticating and granting permission, the user is redirected back to your app with a one-time use code.
- The code is exchanged for an access token that provides all of the requested data and functionality.
To enable OAuth for Google and Microsoft 365 via hosted authentication, you need to create an application for each provider and supply the necessary credentials to Nylas via the developer dashboard. Take a look at our provider integration guides to learn more.
Single sign-on is automatically supported when using OAuth flows to authenticate accounts. All other email providers, including IMAP providers, are supported with no prior configuration.
Native Authentication
Native authentication is for developers who want to customize the login experience through Nylas to match their application, which can completely hide that you’re using Nylas on the backend from your users.
The native authentication guide walks you through how to get started with this option.
Nylas Token Lifecycle
Nylas access tokens don’t expire, so we recommend that you revoke unused tokens whenever you re-authenticate an account, to minimize the number of active tokens. See the Security section below for more details.
Security
Nylas handles a lot of sensitive information, so we’ve built our platform from the ground up with security in mind. We implement strict access controls and auditing to ensure we’re doing everything we can to protect sensitive user data and credentials. A lot of the heavy lifting is handled by us, especially when using our Hosted Authentication, which prevents you from directly handling or storing credentials or OAuth tokens for accounts.
When you integrate with Nylas, you gain access to some of this sensitive data via Nylas access tokens. Security of Nylas access tokens is critical for your application because the tokens grant access to all of your end-users’ account data via the Nylas API.
Storing Secrets
If you’re operating in a cloud environment, there is likely some sort of secret management service available to you. Some examples would be:
When using Nylas’ Hosted Authentication, the secrets you should focus on protecting are:
- Nylas client ID and secret
- Nylas access tokens
- Google Cloud application OAuth client ID/secret
- Microsoft 365 application OAuth client ID/secret
If you’re using Native Authentication, you need to protect all of the above secrets and:
- User passwords
- OAuth refresh and access tokens
If you aren’t using a secret manager to store these values, ensure you are encrypting them at rest and in transit. All requests to Nylas must be made over an encrypted TLS connection (HTTPS), and we don’t recommend sending these outside your infrastructure. If you must transmit these secrets, make sure you’re using an encrypted connection.
Many databases have encryption options built in, so you don’t have to implement encryption of these secrets on your own. If you do decide to encrypt the secrets in your application code, use a well-known library like libsodium or a known-secure library included in your programming language’s standard library.
We also don’t recommend integrating Nylas on the client side of your application.
Revoking Tokens
It is good practice when re-authenticating or cancelling accounts to revoke the existing Nylas access tokens using our revoke tokens endpoint to reduce your attack surface. At any given time, your application should only hold one access token per account.
Storing User Data
If your application will store sensitive data from the Nylas API on your servers, you should implement some disk and/or database-level encryption so all of this data is encrypted at rest. Any time any of this data is in transit, it’s also important to ensure the data is encrypted, which usually can be achieved simply by using TLS connections.
Resources
These key management and cryptographic storage cheat sheets will give you a good overview of how you can protect Nylas client secrets and access tokens.
The guide to encryption key management is an easy to follow high-level guide on the basics of encryption that will help you understand the concepts required to build a secure application.
Some general security advice we can give is that you should double-check any libraries or encryption algorithms you use to ensure they’re actually secure. Libraries for cryptography can have vulnerabilities in their implementations, even though they’re based on a secure algorithm. This applies to bindings for different languages as well because most encryption libraries are implemented in low-level languages like C/C++.
If you’re concerned that you don’t know how to properly secure these access tokens and your application client secret, let us know and we can provide guidance.
Webhooks
Nylas supports multiple ways of tracking changes to accounts in real time. Webhooks are the recommended way to receive notifications of changes to any objects, e.g. new messages in a mailbox, because they are lightweight and scalable. Webhooks use a push notification protocol to notify you of changes, rather than you requesting the latest changes from Nylas.
Nylas implements webhooks by sending an HTTP POST request to a URL that you specify when creating your webhook configuration. When you receive a webhook notification, your application then fetches details of the object that changed from the Nylas API.
It’s important to understand that the term webhook is used for both:
- The configuration representing what data changes send webhooks and where to send the data
- The notification that is sent to the configured location when a change is detected on an account
Webhooks can be configured manually via the Nylas developer dashboard or programmatically via the Webhooks API . The reference docs cover the endpoints to manage webhooks, the webhook triggers available, and the structure of webhooks payloads. This is also the single source of truth for different values that Nylas uses, like our request timeout threshold, or our retry policy.
SDK Examples
We have code examples in our SDK repositories for handling webhooks. You can build off these examples, or use them as a reference to get started on your own implementation.
Scalability
It’s important to have scalability in mind when building infrastructure to handle webhooks. If your webhooks endpoint takes too long to handle incoming requests, it will hit the timeout threshold, causing the request to fail. Nylas uses exponential backoff to retry sends if the first attempt fails, but after a certain number of attempts, the webhook notification will be marked it as failed and no further attempts will be made.
We recommending implementing asynchronous processing on your webhooks endpoint and using a queueing system that you can send the notifications through to be processed by a separate service. This will allow the endpoint to remain responsive and gracefully handle bursts of webhooks that can occur when a large number of changes are made, or when new accounts are connected. Fetching data for the object referred to in the webhook notification would be done in the service consuming events from this queue, so it doesn’t block your endpoint response.
Another thing to keep in mind is the ability to add new webhooks servers behind a load balancer as you scale. This also opens the door to providing high availability on your webhooks receiving system.
Ordering
Webhooks aren’t guaranteed to be delivered in order, for several reasons, including internal architecture, external provider behavior, and transient endpoint errors. Most of the time, the order of webhook events will be consistent with the order of events on the related objects, and you’ll see an object get created before it gets updated.
However, your application should be able to handle the rare cases where you receive an updated
or deleted
trigger before you receive a created
trigger for an object. Since the webhook notifications don’t send the actual objects, you can handle this logic when fetching the latest data from our API.
Historical Data
When Nylas starts syncing an account, we sync new data and historical in parallel. This means you'll receive webhooks for historical and new data at the same time until the account is fully synced. If you want to differentiate between new mail and past mail, the linked_at
field included in the accounts endpoint can be compared to the date
field on the message objects. If the date
is less than the linked_at
time, then the message is historical.
Message & Draft Updates
At this time, Nylas does not have a trigger available to send webhook events when drafts are created or updated, or when messages are updated. To track this type of change, we recommend adding delta tracking to your application.
Deltas
Deltas are another way of tracking changes to accounts in real time. We recommend using webhooks over deltas because deltas are slightly harder to scale and require you to keep persistent HTTP connections to Nylas.
The Deltas reference on our delta endpoints provides an introduction and guide on using this method.
In addition to regular HTTP requests that return immediately (which usually aren't the most efficient for deltas), there are two other connection options supported by Nylas for delta endpoints:
- Streaming: receive multiple changes in the same request, only finishing the request when the timeout is reached or the connection is closed.
- Long polling: receive the latest changes in a single request, holding the request open until a batch of changes is delivered, and then closing it. When using long-polling, you need to start a new long poll request every time there are changes, instead of when the timeout is reached.
Deltas from Nylas include the entire objects that changed in the response, rather than requiring a subsequent fetch of data as webhooks do, so you can send them somewhere to be processed immediately. This also means that a newly-connected or busy account will result in a lot of data being returned over deltas.
Error Handling and Monitoring
Even though Nylas has a highly available and robust API, there’s always a chance that you’ll see errors. Most of these are probably transient, so retrying with exponential backoff will yield success. The Nylas sync engine implements retries and has robust error handling behind the scenes to ensure incidents or transient errors on the providers don’t require any intervention on your part.
The different types of errors to be aware of with your Nylas integration are:
- Invalid Credentials - when an accounts OAuth token expired, was revoked, or their password changed
- API errors - when your application makes a request to the Nylas API and you get a non-200 HTTP status code response or a network error
- Account sync errors - persistent errors that prevent our sync engine from getting the latest changes from the provider or writing new changes to the provider
Invalid Credentials
Once in a while, users need to be re-authenticated to Nylas in order for us to connect to their email provider. When and how often this happens is out of our control because it depends on the provider’s policies. The best way to think about how often or randomly this can happen is if you have a Google or Microsoft account that gets signed out of your browser. If users authenticated using their username and password, this will only be required if their password changed.
When this happens, you can still use the Nylas access token to access data already synced, but no new data can be retrieved from the provider until they are re-authenticated.
You can determine an account’s credentials are invalid via the account.invalid
webhook trigger or by regularly querying our Account Management endpoints. You should prompt the user to re-authenticate as soon as the account is flagged by Nylas as invalid credentials status.
API Errors
Nylas follows the HTTP standard for status codes, so we return a 200 success when requests complete without error. Other status codes, like 4xx or 5xx indicate there was an error. Our SDKs will raise exceptions when requests aren’t successful, but if you’re making your own HTTP requests you might need to check status codes in your code. We return a consistent JSON object on errors with a message
field that provides details about the error. You can view more information about API errors in the developer dashboard as well. A lot of the time these are probably transient, so retrying with exponential backoff will yield success. Here’s our reference on different errors we might return.
Account Sync Errors
Nylas syncs accounts automatically behind the scenes, but there are rare times where we’ll encounter persistent errors that prevent us from getting the latest changes from the provider. We surface these errors through webhooks or the account management endpoints. The status of these accounts will be account.stopped
or account.sync_error
.
The steps to fix a stopped account are:
Step 1: Restart Sync
-
When you get an
account.stopped
oraccount.sync_error
status, create automation to immediately restart the customer's sync. We only keep logs for 2 weeks, so it's important we are made aware asap if reauth and troubleshooting do not solve sync issues. -
You can use the code below.
curl -X POST -u YOUR_APP_SECRET https://api.nylas.com/a/YOUR_APP_ID/accounts/ACCOUNT_ID/downgrade
curl -X POST -u YOUR_APP_SECRET https://api.nylas.com/a/YOUR_APP_ID/accounts/ACCOUNT_ID/upgrade
If you tried this and it didn't work, go to Step 2.
Step 2: Reauth Account
- When you get a Stopped Sync status, create automation to immediately ask the customer to reauth.
- Send them an email asking to reauth
- Send them an in-app message asking them to reauth
- We recommend you revoke their token as well.
- Wait for the user reauth.
- Once the user has reauthed, Check to see if the account is in Running or Partial
- If running, then the issue is fixed
- If partial, then wait to see what happens. Partial is temporary and will transition to Running or Stopped.
The user reauthed but the Stopped Sync persists. Go to Step 3.
Step 3
Steps 1-2 are unsuccessful, contact support (or your sales rep if you aren’t a customer yet).
- Check Sync logs within your Nylas Dashboard.
- Go to dashboard.nylas.com
- Click on Accounts on the left side > Select the account that is experiencing the Sync issue
- Look at the Sync Logs and take notice of what the error is. Does the error indicate anything you can try on your side?
- Ask the customer if they changed any setting on their side.
- Contact [email protected] with the information you got from the customer and the troubleshooting you did on your end.
- Providing the following information will help us troubleshoot your issues as quickly as possible. Please including the following details in your email to [email protected]:
- Account-ID
- Message-ID (if applicable)
- Timestamps (if applicable)
- Any errors you're seeing
- Any errors your end-user is seeing
- Include any code (if applicable)
- Any troubleshooting you've done so far
- Any details that will help us reproduce the error (if applicable)
Rate Limiting
Nylas implements rate limiting solely to protect the platform from single accounts affecting the reliability or performance of other accounts. We have generous rate limits so that our API will handle any use case our customers can think of. Our API returns a 429 HTTP status code when you hit our rate limiting, so it’s important to implement exponential backoff for each account if you encounter these.
Here’s our docs on Nylas’ rate limiting that have the technical details.
There are some scenarios that we are subject to the providers’ rate limits:
- Sending email - Nylas uses the provider to send email using the authenticated email account, so different accounts have different sending limits. Most people don’t hit these limits.
- Updating/Creating objects - these are unlikely to get hit because they are in the 10 seconds of thousands per-account daily
/search
endpoint - Nylas proxies searches on this endpoint to the provider, but it’s highly unlikely you’ll hit these limits since they are read-only requests- Message attachments, raw mime, contact pictures - Nylas caches these items for 7 days internally in Amazon S3, any requests for this data after this period will require Nylas to fetch the data from the provider
Nylas Status
Nylas follows industry best practices with regards to reporting on incidents as soon as we identify problems. We do this through our status page, where you can track ongoing incidents and get real time updates on the progress.
Instrumentation
Having instrumentation for a production application to track the health and status helps you be proactive to detect and resolve issues before it impacts your customers. Nylas tracks many different metrics across our platform 24/7 and alerts an on-call engineer in case of any irregularities.
Nylas is a core part of a lot of our customers’ applications, so we recommend tracking any errors with your Nylas integration as well as with your own application to detect any problems. An example would be tracking the success rate of your requests to Nylas and alerting in case you fall below a certain threshold.
We recommend keeping logs of any errors you encounter with Nylas to help debug issues. Most applications have logging already, so this should serve as a reminder.
Missed Webhooks
In the case that your webhooks server is failing for long periods of time, Nylas will mark your webhook as failed. See our docs for receiving webhooks for details of these periods and how we notify you. This means we will stop attempting to send webhooks for your application until the problem is remediated.
Any missed webhooks during this period can be caught up (within 14 days) using our delta endpoints.
Client-Side Integration
Nylas doesn’t recommend integrating to our API from the client-side (i.e. a web browser) because you need your client ID, client secret, and Nylas access token to make requests. These are very sensitive secrets, especially the Nylas access token, because it grants access to an account’s email, calendar, or contact data. Protecting these secrets from attackers is hard to do, so without a lot of care, you could be vulnerable to CSRF or XSS attacks.
A quick read that explains the complexity of this topic:
Another reason we recommend against integrating client-side is because browsers have ephemeral state, so if your client application loses the Nylas access token, they’ll be required to re-authenticate to get a new Nylas access token. This leads to having a bunch of tokens with access to the user’s account data in limbo as well as a poor user experience.
The final reason not to implement your Nylas integration client-side, is that it’s virtually impossible to receive webhooks to stay up-to-date on the latest changes to accounts.
Account Lifecycle
Accounts in Nylas all have a sync status to indicate whether we’re able to get the latest changes or not. Nylas tracks these account states internally for our engineering team to track down errors before customers notice, but it’s also important for you to monitor the accounts you have connected to Nylas to escalate any issues to support.
Our docs on sync statuses are:
The Error Handling section of this guide details the steps you should take to handle accounts that aren’t in an up to date state.